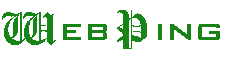
The CGI++ Class Library - Technical Overview
This is a summary overview, and is by no means comprehensive.
For details, please refer to the source code.
Contents
- Methods of Class CGI
- Methods of Class FORM
- The Form Elements Class Hierarchy
- Methods of the Form Elements
- Accessory Modules: FileStream and Escape
- Accessory Modules: Container Classes
CGI derives from FORM, which derives from Map. Hence the methods of
these classes are available.
Initialisation
form()
This method reads and parses any <FORM> data. It supports:
- GET and HEAD: bog-standard decoding of QUERY_STRING
- POST: decoding of form data with content-type
application/x-www-form-urlencoded (the standard) and multipart/form-data
(Netscape's multipart forms, required for the file upload function).
If an unknown Content-Type is supplied, the form data will be placed
(raw, unprocessed) in a buffer
_body
available to the programmer.
- PUT, DELETE, OPTIONS, TRACE: Support is provisional, as there is no
standard for a CGI interface to these methods. Decodes data from
QUERY_STRING (if any). If an Entity Body is supplied, it is
decoded as for POST.
- (null) Assumes you are in debugging mode, and permits you to enter
FORM and/or ENVIRONMENT data interactively.
- (anything else) Assumes an HTTP/1.1 Extension method, and behaves
as with the HTTP/1.1 methods.
Saving and Restoring State
saveto(filename), readfrom(filename)
Save/Restore the contents of a FORM to/from local disc. The saved format
is URLencoded.
Accessing Environment Variables
Direct Access
cenv(name), env(name)
Return the value of environment variable name, as char* or String respectively.
Raw Environment Variables
Methods returning Strings are provided for:
- All CGI Environment Variables
- All HTTP/1.1 General Headers
- All HTTP/1.1 Request Headers
- All HTTP/1.1 Entity Headers
- Netscape Cookies
Note the current standard deployed on most of the Web is HTTP/1.0,
which is a subset of HTTP/1.1. To use CGI++ with HTTP/1.0, simply ignore
the extra methods.
Parsed Environment Variables
Only a few are supported in this release:
- "accept*" and simple "if*" headers: will tell you if a given value is requested
- cookies: will parse KEY=VALUE pairs
DIY Parsed Variables
- key_val
- finds the value of a given key
- word_match
- finds whether a word is matched, ignoring partial words
(so word_match("foo.bar","foo") returns true while word_match("foobar","foo")
is false)
Debugging Functions
- dump_as_text
- Shows all CGI and HTTP Environment Variables and Form Data.
The format is plain text, suitable for interactive debugging or
cut-and-paste from a web browser.
- dump_as_html(action, method, enctype)
- Similar to dump_as_text, but in addition renders all form elements.
This produces a live form, which can be submitted back to the CGI
(provided of course it includes at least one submit button or imagemap).
The arguments are the attributes of HTML <FORM>.
Only
action
is required.
Defining a FORM
define(int, ...)
arguments are an int (number of elements to define) and the element
definitions, each of which is a char*. Arguments take the form
"name:type:value:size1:size2"
Example:
"foo:textarea:some text here:4:40"
defines an element "foo"
which is a textarea with 4 rows and 40 columns, whose initial
contents is "some text here".
Fields not relevant to an element may be left blank; for example
a hidden field, SELECT field or radio group has no "size" attributes.
See the form element definitions for detail.
set_template(char*)
Defines a single element, in the manner described above.
set_delimiter
Allows you to change the character used to parse element definitions
(default ':' as shown in the discussion above)
Printing a FORM
- start(action, method, enctype)
- Opens a new HTML FORM
- render(element)
- Prints a form element as HTML, set to its current value
- submit(label)
- Prints a submit button displaying the label
- reset(label)
- Prints a reset button displaying the label
- end()
- Closes the form.
Debugging
- dump_as_text, dump_as_html
- These are the FORM parts of the CGI dump functions described above.
Accessing Form Elements
Accessors are derived from the Map parent class. In particular, a FORM
or CGI is an associative array of elements, indexed by name, and both
of the following work:
form[name] = element ;
element = form[name] ;
There's some extra type checking in the Form Elements, which will help
avoid silly things like
form_select s = form["abcd"]
where form["abcd"] is not of type form_select.
Iterating over Form Elements
Iteration is a function of the parent Map class, using the standard
iterator Pix
and a C-style loop:
for (Pix x = form.first() ; x ; form.next(x)) {
// do something with form[x] ;
}
The base class for all form elements is form_element,
and there is a pretty standard object-oriented hierarchy. User-level
form elements are:
- form_input - an ordinary text input field
- form_hidden - a hidden field
- form_password - a password field
- form_textarea - a textarea
- form_radio - a radio group
- form_select - a popup menu
- form_select_multiple - a multiple-choice menu
- form_submit - a submit button
- form_checkbox - a checkbox
- form_imagemap - an imagemap
- form_file - a file upload field
(A form_imagemap defines three elements: one "master" and two of
type "form_imagemap_coord". This is required when a form is read,
to prevent "[name].x" and "[name].y" being tagged as unknown elements -
which default to type "form_input".)
The generic class used to hold form elements in a Map is FEP
(Form Element Pointer).
Constructor: Elements have constructors appropriate to their contents.
In addition, every element has a constructor taking a FEP argument, and
performing type checking. This permits the
element = form[name]
notation.
Setting Values
set(String)
Set a the value - behaviour differs between the elements:
- checkbox - OFF for null string, ON otherwise
- select_multiple - adds the argument to the Bag of selected values
- imagemap - sets the URL (relative or absolute) of the image source file
- others - sets the value of the field
Display Methods
CDATA& as_html() - value suitable for including in an HTML page
String& as_text() - value in plain text
char* as_longtext() - Plain text (multipart forms).
This overcomes the 32K limit on the String class (and hence on the first
release of CGI++).
CDATA& render(name) - Fully functioning HTML rendition of the element.
Other
String& encode(name) - returns URLescaped element
String& filename(String&) - Set/read filename for file upload parameters.
Additional methods of particular elements
form_input, form_password, form_textarea, form_file,
form_imagemap
have two optional parameters defining their size when rendered.
These are extra arguments to their constructors.
form_radio, form_select, form_select_multiple
each include a map of the values displayed in HTML when rendered against
those returned to CGI when selected. This is accessed by the [] notation:
option["option1"] = "The first option"
and other methods of Map.
form_checkbox
has binary method checked()
form_select_multiple
Selected values are a "Bag"; extra accessor methods are:
- unset(String) - opposite of set()
- clear() - unset all values
- value() - returns the whole bag
form_imagemap
x(), y() - integer values of the coordinates
FileStream
Istream, Ostream
define input and output File streams, with builtin locking and error
handling. They are derived from ifstream and ofstream respectively,
and so support all normal stream operations. Constructors for both
classes take the filename as argument, and open and lock the file
(opening uses a safe bare-open/lock/attach-stream regime).
Destructors unlock and close the file.
Escape
Defines functions www_escape, www_unescape
and html_escape.
These take argument String&
and return String&
.
Normally they will
modify the string in situ, but if passed a const argument they
will create and modify a copy.
Container classes for Map and Bag
are used as Base classes for the CGI++ classes. These are generated
using the GNU genclass utility.
These may be replaced by equivalent STL classes in a future release.
The public interface (as described above) should not be affected.